In Part 1 of our post for Getting Started with MS Graph, we had covered the basics of how to complete an app registration in Azure.
In this part 2, we will cover how to connect to the MS Graph REST API and provide some samples on how to do some cool stuff! Let's go!
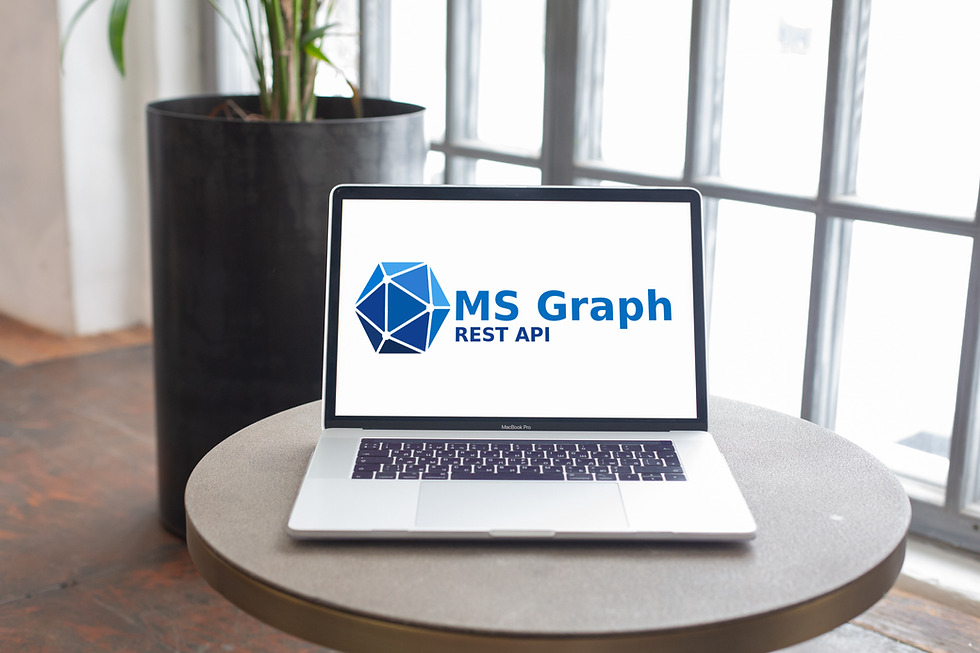
Obtaining the Authentication Token
In order to connect to the MS Graph REST API we created in Part 1 we need an Authentication Token. To acquire this token, we will leverage the Microsoft Authentication Library (MSAL).
“The Microsoft Authentication Library (MSAL) enables developers to acquire tokens from the Microsoft identity platform in order to authenticate users and access secured web APIs. It can be used to provide secure access to Microsoft Graph, other Microsoft APIs, third-party web APIs, or your own web API. MSAL supports many different application architectures and platforms including .NET, JavaScript, Java, Python, Android, and iOS.”
We won't cover what MSAL is in detail but if you need or want, you can check out additional detailed information regarding MS Graph Authentication from Microsoft.
The Authentication Token will allow us to construct the Authentication Header that is required when querying the MS Graph REST API.
A PowerShell module is available that wraps MSAL.NET functionality into PowerShell-friendly cmdlets. This module is called “MSAL.PS”. For additional detailed information regarding this module refer to the MSAL GitHub and PowerShell Gallery for the MSAL Module.
For the purpose of this demo I will be using PowerShell Core. MSAL.PS will also work on Windows PowerShell.
To start, check to see if you have the “MSAL.PS” module already installed.
Get-InstalledModule | Where-Object{$_.Name -eq "MSAL.PS"}
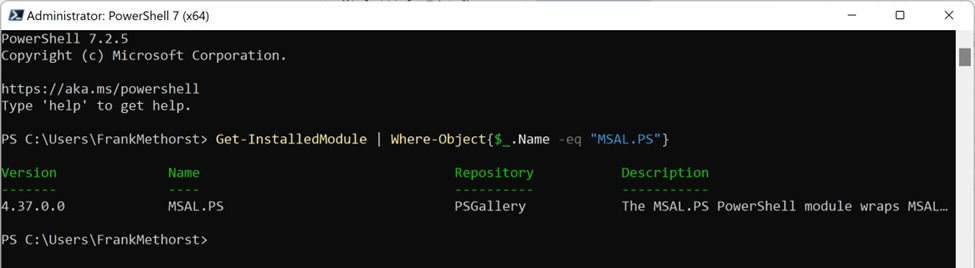
If not, install it.
Install-Module MSAL.PS
For detailed instructions on how to install the MSAL.PS module, refer to GitHub.
With the MSAL module successfully installed, let’s create the Authentication token for an interactive user session with the MS Graph API we created in Part 1.
You will need the following information:
Application ID – You can find this on the Overview page of the App Registration you created in Part1. Tenant ID - You can find this on the Overview page of the App Registration you created in Part 1.
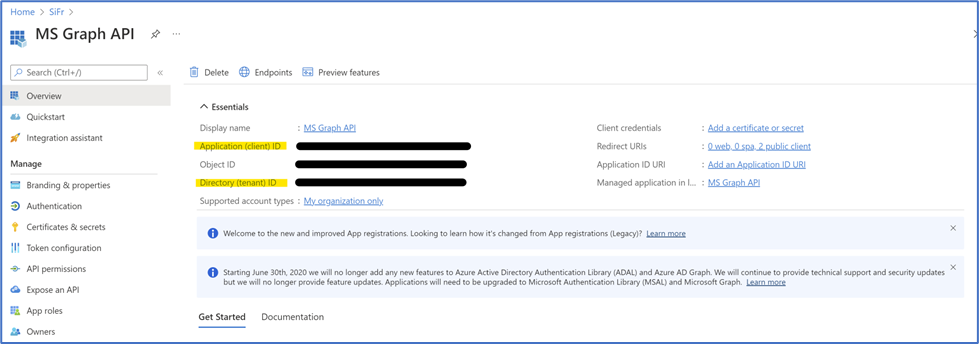
Scope – refers to the permissions granted during authentication. For instance, you may only need read access for users in Azure AD.
For this particular blog demo, our scope would be "User.Read.All".
If you needed to write user attributes in Azure AD our scope would be “User.ReadWrite.All”.
As you might guess Devices follow the same convention “Device.Read.All” etc.
Additionally broader permissions are available if multiple object types need to be accessed, for instance, “Directory.Read.All”.
For additional detailed Information on MS Graph permissions, check out the Microsoft Graph Permission Reference.
Here is an example of permissions associated with a specific action (Get User) : Get a user - Microsoft Graph v1.0 | Microsoft Docs
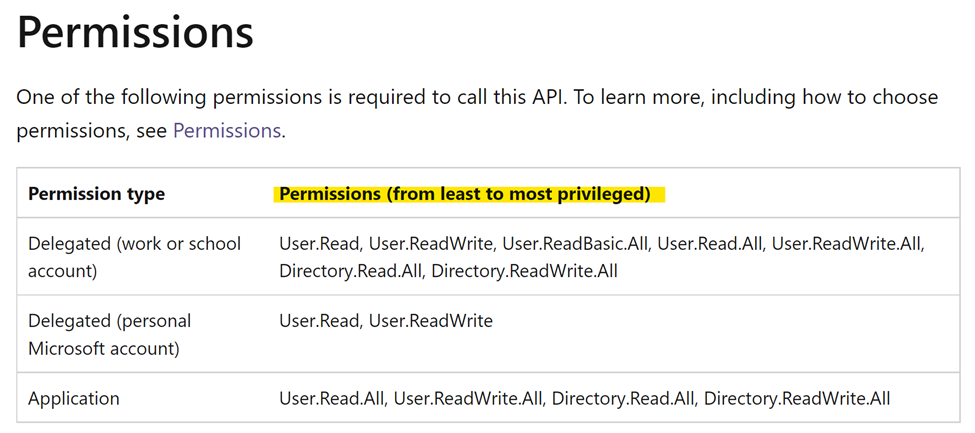
In PowerShell add the three required parameters to variables:

Run the following command:
$MSALToken = Get-MsalToken -ClientId $ApplicationID -TenantId $TenantID -Interactive -Scope $Scope
Assuming the parameters are correct you should see a Modern Authentication prompt. Enter credentials that have sufficient rights to do the thing your automation needs to do.
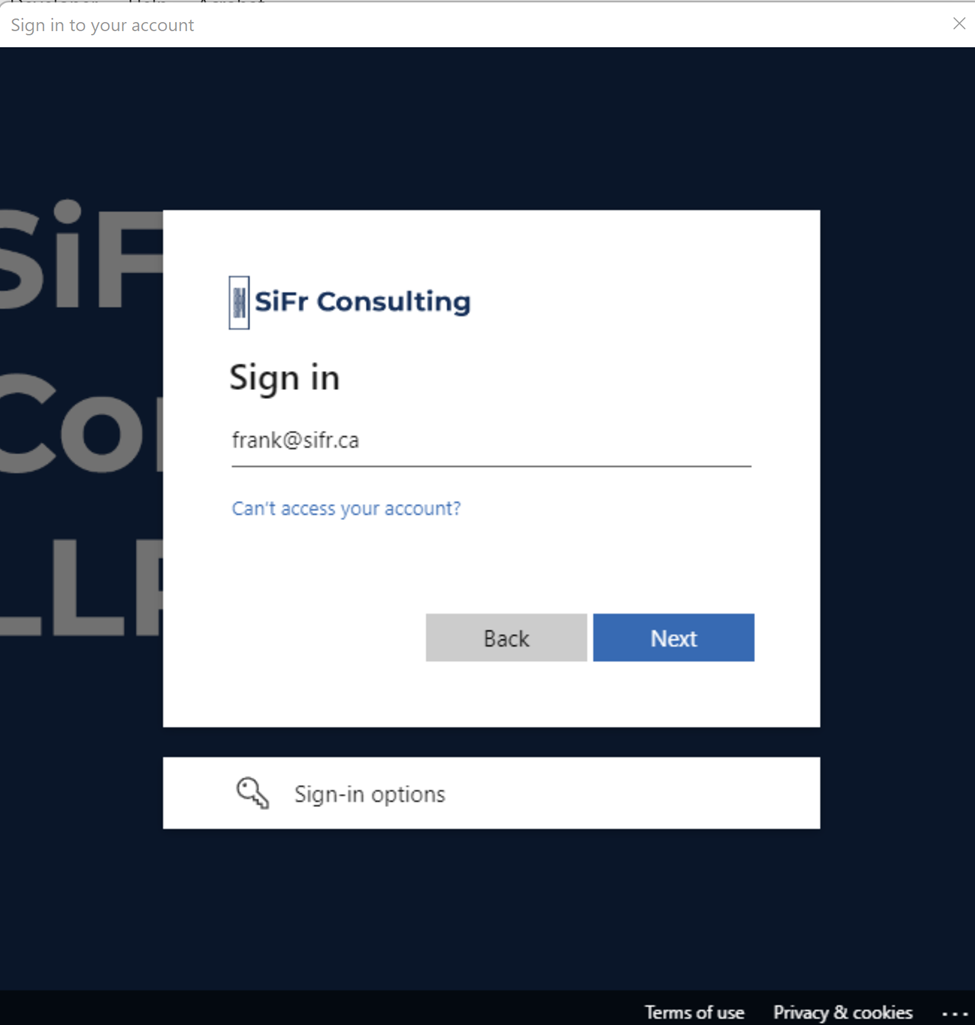
You will be prompted to approve the permissions you specified in the Scope parameter.
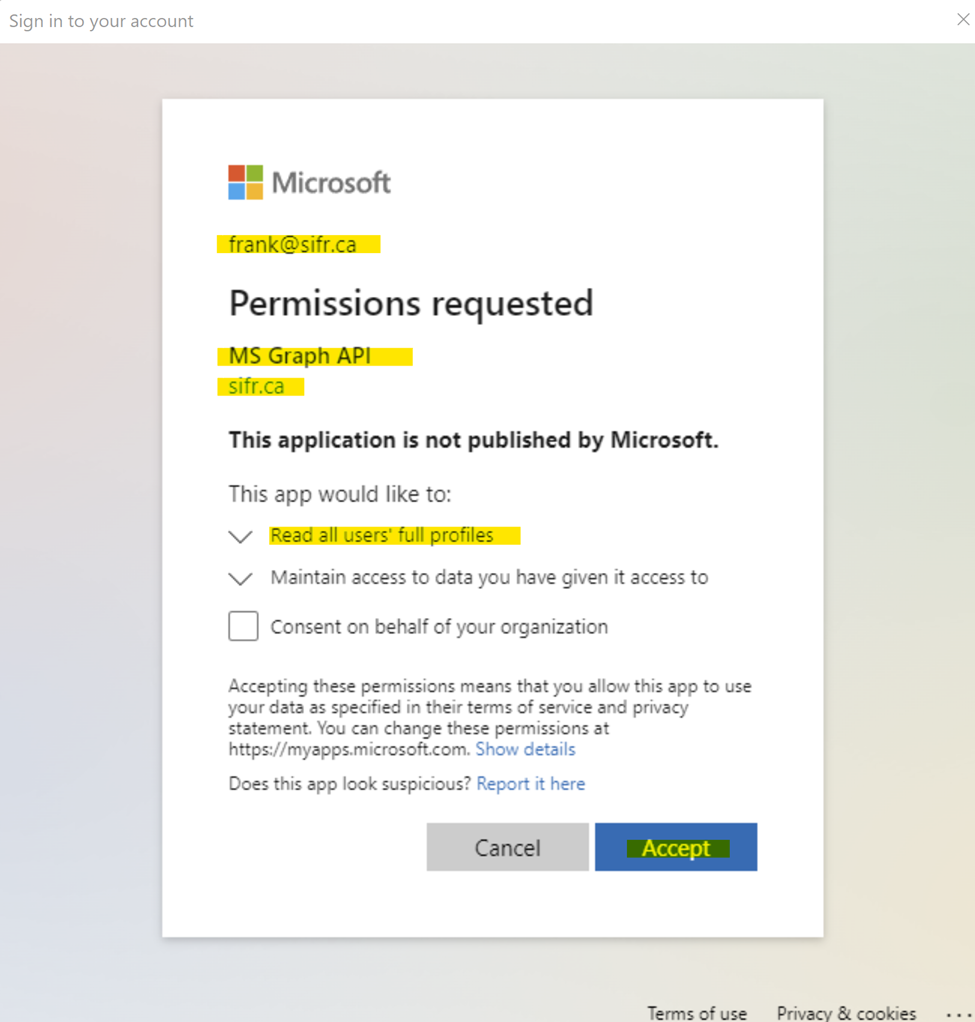
NOTE: If you tick “Consent on behalf of your organization” you will be authorizing delegated access for other users in your organization to access this data. If you want to keep this locked down, leave it unticked. You will be prompted each time you access the API.
After successfully authenticating your $MSALToken variable should contain the MSAL Token object. The properties and methods of interest include (highlighted):
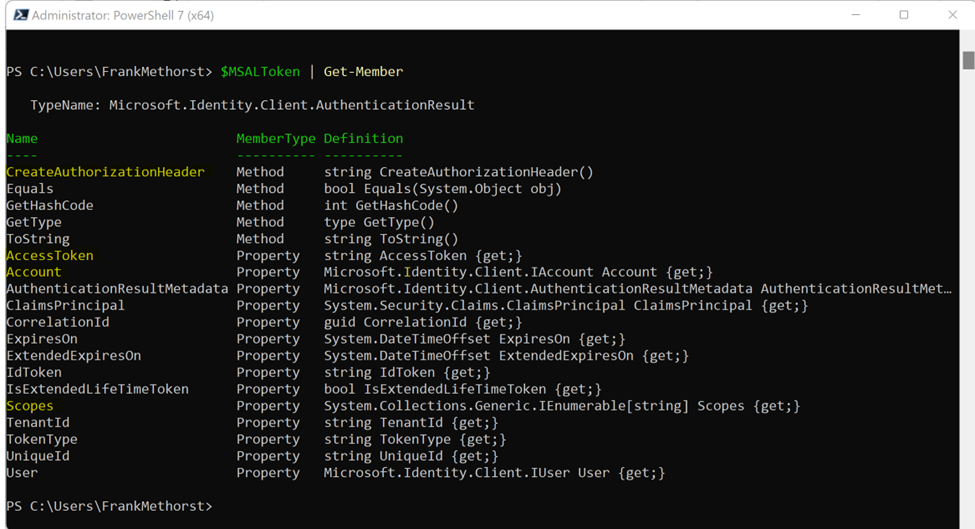
Constructing the Authentication Header
Next we are going to construct the Authentication Header that is required each time the MS Graph REST API is queried.
$authHeader = @{'Authorization' = $MSALToken.CreateAuthorizationHeader()}

Because our granted API scope was "User.Read.All", our Auth Header will allow the automation to read all properties of all Azure AD users. Effectively, allowing us to query Azure AD users.
For fun, let’s do a quick query and return some users:
$URI = 'https://graph.microsoft.com/v1.0/users'
$Response = Invoke-RestMethod -Uri $URI -Headers $authHeader -Method Get
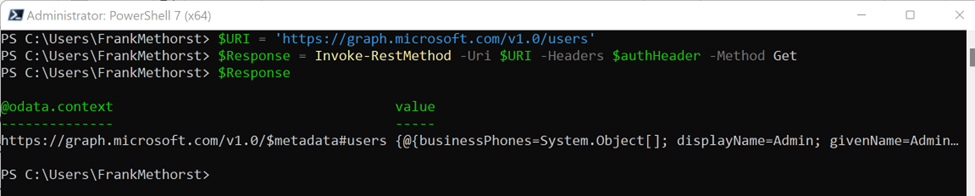
The information you are interested in is returned in the value property.
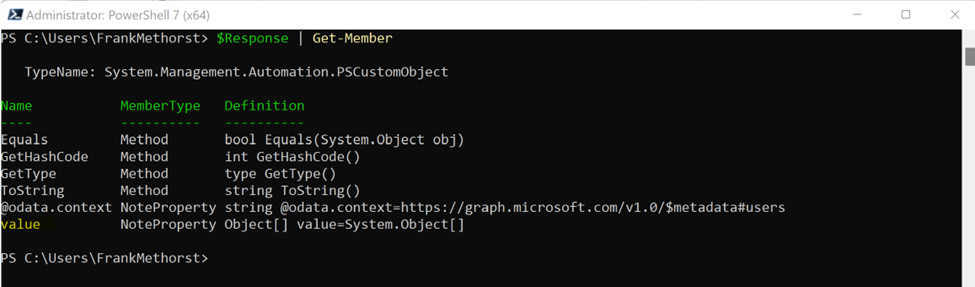
$Response.value
Here are the default values that are returned:
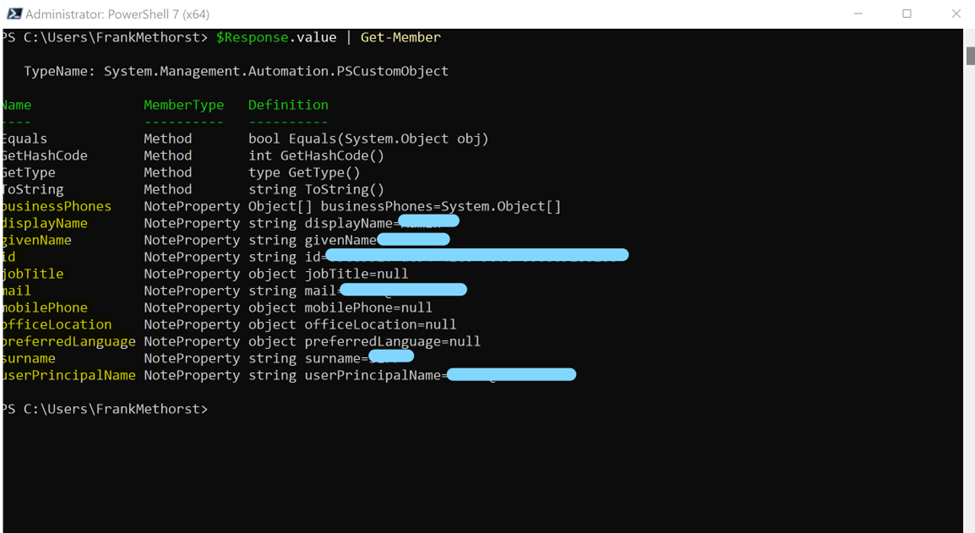
You may have noticed a number of limitations on the information returned, including a limit on the number of records and which attributes are returned. In Part 3 of the series I will show you how to deal with these issues and discuss MS Graph API queries in detail. Be sure to subscribe to be notified when new posts become live.
Hope you've found this post helpful. If you did, please share it with your admin friends so they can benefit too! Sharing is caring.
If you need some additional support on automation, we're very good at this stuff. So contact us to see if we can help support your business goals.
The content on this web site is provided for general information purposes only and does not constitute professional advice. Users of this web site are advised to seek specific technical advice by contacting SiFr or their own IT resource regarding any specific technical issues. SiFr does not warrant or guarantee the quality, accuracy or completeness of any information on this web site. The articles published on this web site are current as of their original date of publication, but should not be relied upon as accurate, timely or fit for any particular purpose.
This web site may contain links to third party web sites. Links are provided for convenience only and SiFr does not endorse the information contained in linked web sites nor guarantee its accuracy, timeliness or fitness for a particular purpose.
SiFr is a Microsoft Partner.
تعليقات